Minecraft Modding Tutorials
Learn the basics, explore advanced techniques, and create mods that transform your Minecraft world. These step-by-step tutorials are designed to make getting into modding Minecraft a bit easier!
Creating Items ‐ MultiLoader 1.21+
Creating new items is one of the key starting points to many mods. In this tutorial, we will create a basic item with a model and texture.
Before you continue with this part of the tutorial, please ensure that you have followed the tutorial for Setting Up RegistrationUtils.
Creating the Item Registry
What you will first want to do is create the class where we will register our items. For the purposes of this tutorial, I will name this ItemRegistry and place it in another package called registry, but feel free to call this whatever, and put it wherever, you like.
In the package structure, it will look like com.example.examplemod.registry.ItemRegistry.
In this class, we will first define an empty, public static void function called init, and we will use this later as a point of loading the class. I use the term init as it refers to the initialisation of the class and its contents, but again, you can name this whatever you like.
Next, we will define a RegistrationProvider as follows using the Item registry:
public static final RegistrationProvider- ITEMS = RegistrationProvider.get(Registries.ITEM, Constants.MOD_ID);
This will be used to add our items to the Item registry, and we will get to where we will use this elsewhere in a little bit.
Before creating the Item field, we will first define a function in this class called getItemProperties, which will be used later in the tutorial.
public static Item.Properties getItemProperties() {
return new Item.Properties();
}
After this, we will now create a new item, which in this tutorial we will call "New Dirt". To do this,
create a new public static final field of the type RegistryObject
public static final RegistryObject NEW_DIRT = registerBlock("new_dirt",
() -> new Block(BlockBehaviour.Properties.ofFullCopy(Blocks.DIRT)));
Here we are copying all the block properties from the vanilla dirt block, but you can use the .of()... builder pattern to assign other properties like jump factor, light level, explosion resistance, etc.
Now what we want to do, is in your init function in CommonClass, you will want to call the init method of your BlockRegistry.
Using this new method, in both your Fabric and NeoForge projects, you will want to go to the ExampleMod classes for each.
Congratulations, you made your first Block! But wait... how will you even be able to see it in game? We'll follow a similar process to creating items but its slightly different.
Creating a Block Model and Defining Block State
First, in your common project, create a new file called new_dirt.json in the resources directory in the following directory: assets/<examplemod>/models/block. Remember to replace examplemod with your own Mod ID if you changed it.
Inside this file, you are going to add the following:
{
"parent": "minecraft:block/cube_all",
"textures": {
"all": "examplemod:block/new_dirt"
}
}
This defines the model using the block/cube_all parent block model from Minecraft's own set of models. The all parameter sets the name of the texture file to use for all faces of the block. Remember to replace examplemod with your own Mod ID if you changed it. You will want to name this file the same as the lowercase name you gave the block in the registry, and also change the name of the block in the textures block if this is also different.
After this, in the same resources directory, you will want to create a 16x16 PNG image file for the block, and place it in the following directory: assets/<examplemod>/textures/block. You will want to name this file the same as the lowercase name you gave the block in the registry.
Next we will create a blockstate file. Create a new json file called new_dirt.json in the following directory: assets/<examplemod>/textures/blockstates. In this file, we will define the blockstates, which allow us to change the state (i.e., model or texture) of the block using its properties. In this case, we are creating just a simple block, so you can use the code below, but more information on blockstates can be found here
Finally, we are going to do two more things. First we are going to change how we do our creative tabs. Create a new class in the registry package and call it CreativeTabRegistry. As with the others, we are going to create an init function and call this in our CommonClass, and we are going to copy over the creative tab code from our ItemRegistry. Next, copy the item registry and rename it to blocks, and make sure you change references of TAB/tab. Remember to remove the existing code from the ItemRegistry or you will encounter errors. Your new CreativeTabRegistry file will look like this:
{
"item.examplemod.iron_stick": "Iron Stick"
}
For any further entries into this language file, you will need to add a comma before every new line. For other languages, you can use the same approach, but the only difference is the name of the language file you enter this into. For other language files, refer to the "In-game" language codes found in the table in the dedicated Language section on the Minecraft Wiki.
And that's it! You should be able to get your new item using the /give <player> <item> [amount] command in game. Replace <item> with <examplemod:iron_stick> or whatever you named your Mod ID and Item.
We all like creative tabs...
I agree, using commands sucks when you can have creative tabs to get your items easier. We will create recipes later in this tutorial series, but for now let's make a new creative tab for our mod.
In the ItemRegistry class, add the following code:
public static final RegistrationProvider<CreativeModeTab>CREATIVE_MODE_TABS = RegistrationProvider.get(Registries.CREATIVE_MODE_TAB, Constants.MOD_ID);
public static final RegistryObject<CreativeModeTab> TAB = CREATIVE_MODE_TABS.register(Constants.MOD_ID + "_tab", () -> CreativeModeTab.builder(CreativeModeTab.Row.TOP, 0)
.icon(() -> new ItemStack(IRON_STICK.get()))
.displayItems(
(itemDisplayParameters, output) -> {
output.accept(IRON_STICK.get());
}).title(Component.translatable("itemGroup." + Constants.MOD_ID + ".tab"))
.build());
The icon function takes an ItemStack as the parameter here with our new item as the icon to display for the tab in the creative mode inventory. The displayItems function allows us to assign the output of what to put in this tab. We use the output.accept method here to add our new item.
You'll notice that there is also a title function. This takes a Component as the parameter, so we have to now add a new entry into our lang file to add this item group. Here's the updated language file we created before:
{
"item.examplemod.iron_stick": "Iron Stick",
"itemGroup.examplemod.tab": "Example Mod Items"
}
And ta-da! You've created your own item and added it to a custom creative tab successfully!
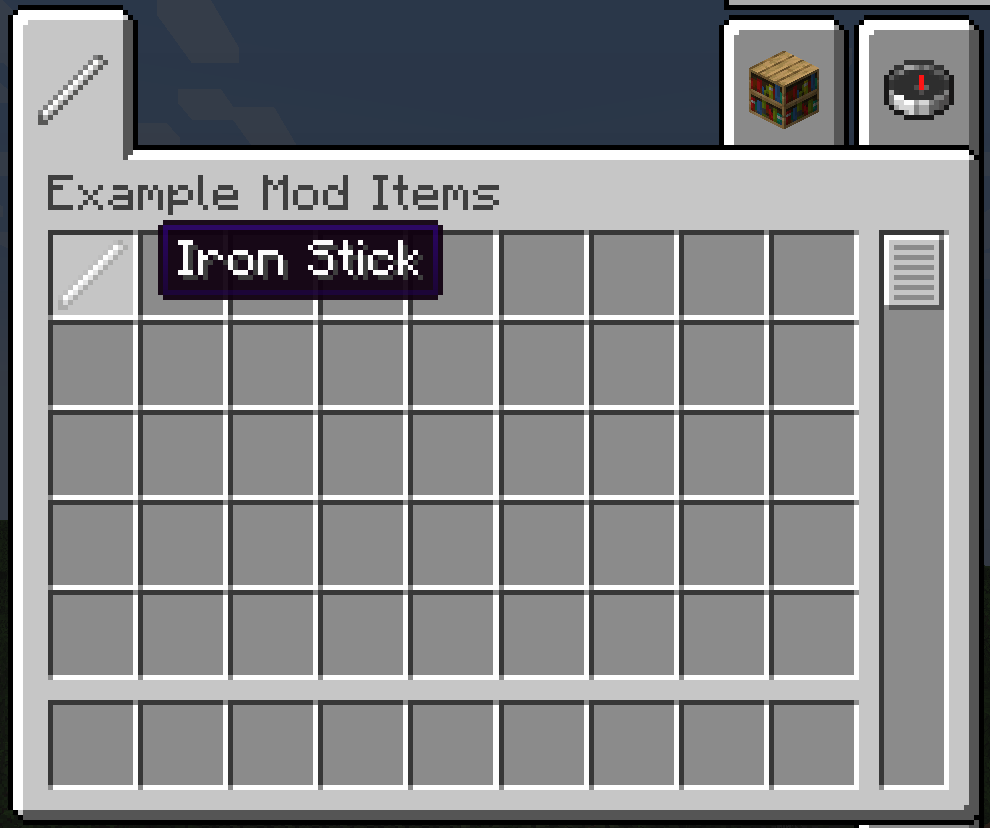
You can find the source for this tutorial here:
View Source